mirror of
https://github.com/pluginsGLPI/example.git
synced 2025-05-04 18:08:42 +02:00
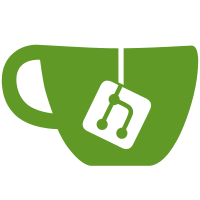
git-svn-id: https://forge.glpi-project.org/svn/example/trunk@202 349b9182-4a13-0410-896f-e5e9767dd1b3
328 lines
9.9 KiB
PHP
328 lines
9.9 KiB
PHP
<?php
|
|
/*
|
|
* @version $Id: HEADER 15930 2011-10-25 10:47:55Z jmd $
|
|
-------------------------------------------------------------------------
|
|
GLPI - Gestionnaire Libre de Parc Informatique
|
|
Copyright (C) 2003-2011 by the INDEPNET Development Team.
|
|
|
|
http://indepnet.net/ http://glpi-project.org
|
|
-------------------------------------------------------------------------
|
|
|
|
LICENSE
|
|
|
|
This file is part of GLPI.
|
|
|
|
GLPI is free software; you can redistribute it and/or modify
|
|
it under the terms of the GNU General Public License as published by
|
|
the Free Software Foundation; either version 2 of the License, or
|
|
(at your option) any later version.
|
|
|
|
GLPI is distributed in the hope that it will be useful,
|
|
but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
|
GNU General Public License for more details.
|
|
|
|
You should have received a copy of the GNU General Public License
|
|
along with GLPI. If not, see <http://www.gnu.org/licenses/>.
|
|
--------------------------------------------------------------------------
|
|
*/
|
|
|
|
// ----------------------------------------------------------------------
|
|
// Original Author of file:
|
|
// Purpose of file:
|
|
// ----------------------------------------------------------------------
|
|
|
|
// Class of the defined type
|
|
class PluginExampleExample extends CommonDBTM {
|
|
|
|
|
|
// Should return the localized name of the type
|
|
static function getTypeName($nb = 0) {
|
|
return 'Example Type';
|
|
}
|
|
|
|
|
|
static function canCreate() {
|
|
|
|
if (isset($_SESSION["glpi_plugin_example_profile"])) {
|
|
return ($_SESSION["glpi_plugin_example_profile"]['example'] == 'w');
|
|
}
|
|
return false;
|
|
}
|
|
|
|
|
|
static function canView() {
|
|
|
|
if (isset($_SESSION["glpi_plugin_example_profile"])) {
|
|
return ($_SESSION["glpi_plugin_example_profile"]['example'] == 'w'
|
|
|| $_SESSION["glpi_plugin_example_profile"]['example'] == 'r');
|
|
}
|
|
return false;
|
|
}
|
|
|
|
|
|
function getSearchOptions() {
|
|
|
|
$tab = array();
|
|
$tab['common'] = "Header Needed";
|
|
|
|
$tab[1]['table'] = 'glpi_plugin_example_examples';
|
|
$tab[1]['field'] = 'name';
|
|
$tab[1]['name'] = __('Name');
|
|
|
|
$tab[2]['table'] = 'glpi_plugin_example_dropdowns';
|
|
$tab[2]['field'] = 'name';
|
|
$tab[2]['name'] = __('Dropdown');
|
|
|
|
$tab[3]['table'] = 'glpi_plugin_example_examples';
|
|
$tab[3]['field'] = 'serial';
|
|
$tab[3]['name'] = __('Serial number');
|
|
$tab[3]['usehaving'] = true;
|
|
$tab[3]['searchtype'] = 'equals';
|
|
|
|
$tab[30]['table'] = 'glpi_plugin_example_examples';
|
|
$tab[30]['field'] = 'id';
|
|
$tab[30]['name'] = __('ID');
|
|
|
|
return $tab;
|
|
}
|
|
|
|
|
|
/**
|
|
* Give localized information about 1 task
|
|
*
|
|
* @param $name of the task
|
|
*
|
|
* @return array of strings
|
|
*/
|
|
static function cronInfo($name) {
|
|
|
|
switch ($name) {
|
|
case 'Sample' :
|
|
return array('description' => __('Cron description for example', 'example'),
|
|
'parameter' => __('Cron parameter for example', 'example'));
|
|
}
|
|
return array();
|
|
}
|
|
|
|
|
|
/**
|
|
* Execute 1 task manage by the plugin
|
|
*
|
|
* @param $task Object of CronTask class for log / stat
|
|
*
|
|
* @return interger
|
|
* >0 : done
|
|
* <0 : to be run again (not finished)
|
|
* 0 : nothing to do
|
|
*/
|
|
static function cronSample($task) {
|
|
|
|
$task->log("Example log message from class");
|
|
$r = mt_rand(0,$task->fields['param']);
|
|
usleep(1000000+$r*1000);
|
|
$task->setVolume($r);
|
|
|
|
return 1;
|
|
}
|
|
|
|
|
|
// Hook done on before add item case (data from form, not altered)
|
|
static function pre_item_add_computer(Computer $item) {
|
|
if (isset($item->input['name']) && empty($item->input['name'])) {
|
|
Session::addMessageAfterRedirect("Pre Add Computer Hook KO (name empty)", true);
|
|
return $item->input = false;
|
|
} else {
|
|
Session::addMessageAfterRedirect("Pre Add Computer Hook OK", true);
|
|
}
|
|
}
|
|
|
|
// Hook done on before add item case (data altered by object prepareInputForAdd)
|
|
static function post_prepareadd_computer(Computer$item) {
|
|
Session::addMessageAfterRedirect("Post prepareAdd Computer Hook", true);
|
|
}
|
|
|
|
|
|
// Hook done on add item case
|
|
static function item_add_computer(Computer$item) {
|
|
|
|
Session::addMessageAfterRedirect("Add Computer Hook, ID=".$item->getID(), true);
|
|
return true;
|
|
}
|
|
|
|
|
|
function getTabNameForItem(CommonGLPI $item, $withtemplate=0) {
|
|
|
|
if (!$withtemplate) {
|
|
switch ($item->getType()) {
|
|
case 'Profile' :
|
|
if ($item->getField('central')) {
|
|
return __('Example', 'example');
|
|
}
|
|
break;
|
|
|
|
case 'Phone' :
|
|
if ($_SESSION['glpishow_count_on_tabs']) {
|
|
return self::createTabEntry(__('Example', 'example'),
|
|
countElementsInTable($this->getTable()));
|
|
}
|
|
return __('Example', 'example');
|
|
|
|
case 'ComputerDisk' :
|
|
case 'Supplier' :
|
|
return array(1 => __("Test PLugin", 'example'),
|
|
2 => __("Test PLugin 2", 'example'));
|
|
|
|
case 'Computer' :
|
|
case 'Central' :
|
|
case 'Preference':
|
|
case 'Notification':
|
|
return array(1 => __("Test PLugin", 'example'));
|
|
|
|
}
|
|
}
|
|
return '';
|
|
}
|
|
|
|
|
|
static function displayTabContentForItem(CommonGLPI $item, $tabnum=1, $withtemplate=0) {
|
|
|
|
switch ($item->getType()) {
|
|
case 'Phone' :
|
|
_e("Plugin Example on Phone", 'example');
|
|
break;
|
|
|
|
case 'Central' :
|
|
_e("Plugin central action", 'example');
|
|
break;
|
|
|
|
case 'Preference' :
|
|
// Complete form display
|
|
$data = plugin_version_example();
|
|
|
|
echo "<form action='Where to post form'>";
|
|
echo "<table class='tab_cadre_fixe'>";
|
|
echo "<tr><th colspan='3'>".$data['name']." - ".$data['version'];
|
|
echo "</th></tr>";
|
|
|
|
echo "<tr class='tab_bg_1'><td>Name of the pref</td>";
|
|
echo "<td>Input to set the pref</td>";
|
|
|
|
echo "<td><input class='submit' type='submit' name='submit' value='submit'></td>";
|
|
echo "</tr>";
|
|
|
|
echo "</table>";
|
|
echo "</form>";
|
|
break;
|
|
|
|
case 'Notification' :
|
|
_e("Plugin mailing action", 'example');
|
|
break;
|
|
|
|
case 'ComputerDisk' :
|
|
case 'Supplier' :
|
|
if ($tabnum==1) {
|
|
_e('First tab of Plugin example', 'example');
|
|
} else {
|
|
_e('Second tab of Plugin example', 'example');
|
|
}
|
|
break;
|
|
|
|
default :
|
|
//TRANS: %1$s is a class name, %2$d is an item ID
|
|
printf(__('Plugin example CLASS=%1$s id=%2$d', 'example'), $item->getType(), $item->getField('id'));
|
|
break;
|
|
}
|
|
return true;
|
|
}
|
|
|
|
static function getSpecificValueToDisplay($field, $values, array $options=array()) {
|
|
|
|
if (!is_array($values)) {
|
|
$values = array($field => $values);
|
|
}
|
|
switch ($field) {
|
|
case 'serial' :
|
|
return "S/N: ".$values[$field];
|
|
}
|
|
return '';
|
|
}
|
|
|
|
// Parm contains begin, end and who
|
|
// Create data to be displayed in the planning of $parm["who"] or $parm["who_group"] between $parm["begin"] and $parm["end"]
|
|
static function populatePlanning($parm) {
|
|
|
|
// Add items in the output array
|
|
// Items need to have an unique index beginning by the begin date of the item to display
|
|
// needed to be correcly displayed
|
|
$output = array();
|
|
$key = $parm["begin"]."$$$"."plugin_example1";
|
|
$output[$key]["begin"] = date("Y-m-d 17:00:00");
|
|
$output[$key]["end"] = date("Y-m-d 18:00:00");
|
|
$output[$key]["name"] = __("test planning example 1", 'example');
|
|
// Specify the itemtype to be able to use specific display system
|
|
$output[$key]["itemtype"] = "PluginExampleExample";
|
|
// Set the ID using the ID of the item in the database to have unique ID
|
|
$output[$key][getForeignKeyFieldForItemType('PluginExampleExample')] = 1;
|
|
return $output;
|
|
}
|
|
|
|
/**
|
|
* Display a Planning Item
|
|
*
|
|
* @param $val Array of the item to display
|
|
* @param $who ID of the user (0 if all)
|
|
* @param $type position of the item in the time block (in, through, begin or end)
|
|
* @param $complete complete display (more details)
|
|
*
|
|
* @return Nothing (display function)
|
|
**/
|
|
static function displayPlanningItem(array $val, $who, $type="", $complete=0) {
|
|
|
|
// $parm["type"] say begin end in or from type
|
|
// Add items in the items fields of the parm array
|
|
switch ($type) {
|
|
case "in" :
|
|
//TRANS: %1$s is the start time of a planned item, %2$s is the end
|
|
printf(__('From %1$s to %2$s :'),
|
|
date("H:i",strtotime($val["begin"])), date("H:i",strtotime($val["end"]))) ;
|
|
break;
|
|
|
|
case "through" :
|
|
echo Html::resume_text($val["name"],80);
|
|
break;
|
|
|
|
case "begin" :
|
|
//TRANS: %s is the start time of a planned item
|
|
printf(__('Start at %s:'), date("H:i", strtotime($val["begin"]))) ;
|
|
break;
|
|
|
|
case "end" :
|
|
//TRANS: %s is the end time of a planned item
|
|
printf(__('End at %s:'), date("H:i", strtotime($val["end"]))) ;
|
|
break;
|
|
}
|
|
echo "<br>";
|
|
echo Html::resume_text($val["name"],80);
|
|
}
|
|
|
|
/**
|
|
* Get an history entry message
|
|
*
|
|
* @param $data Array from glpi_logs table
|
|
*
|
|
* @since GLPI version 0.84
|
|
*
|
|
* @return string
|
|
**/
|
|
static function getHistoryEntry($data) {
|
|
|
|
switch($data['linked_action'] - Log::HISTORY_PLUGIN) {
|
|
case 0:
|
|
return __('History from plugin example', 'example');
|
|
}
|
|
|
|
return '';
|
|
}
|
|
}
|
|
?>
|